Learn how to code a simple trading algorithm in python — Coding Part II
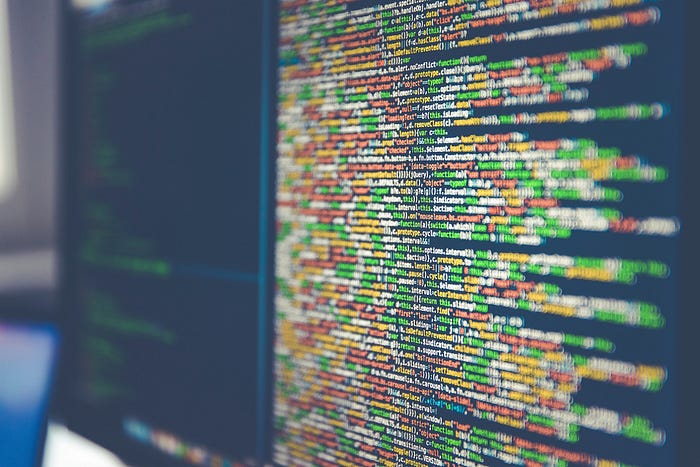
In the following, we learn how to code a simple long/short equity strategy. First some basics what this strategy is using the Tesla Inc as an example:
A long/short equity strategy involves taking both long positions (buying stocks with the expectation that their price will increase) and short positions (selling stocks that have been borrowed with the expectation that their price will decrease).
For example, let’s say a hedge fund is using a long/short equity strategy and is considering the Tesla stock. The fund’s analysts may believe that Tesla’s stock price is likely to increase due to strong sales and positive earnings reports. In this case, the fund may decide to take a long position in Tesla by buying shares of the stock.
On the other hand, the fund’s analysts may also identify another stock that they believe is overvalued and likely to decrease in price. In this case, the fund may decide to take a short position in the stock by selling shares that have been borrowed.
By taking both long and short positions, a long/short equity strategy can potentially generate returns in both rising and falling markets. However, it is important to note that this strategy carries the risk of losses if the stocks held in long positions decrease in value or if the stocks held in short positions increase in value.
Now we know how it works, let’s start coding this strategy in python:
# Import necessary libraries
import pandas as pd
import numpy as np
# Load stock data for Tesla
tesla = pd.read_csv("TSLA.csv")
# Calculate the 50-day and 200-day moving averages for Tesla
tesla["50_day_ma"] = tesla["Close"].rolling(50).mean()
tesla["200_day_ma"] = tesla["Close"].rolling(200).mean()
# Set the initial capital for the strategy
initial_capital = 100000
# Set the initial positions for Tesla
tesla["Position"] = 0
# Iterate through each row in the dataframe
for index, row in tesla.iterrows():
# If the 50-day moving average is greater than the 200-day moving average, take a long position in Tesla
if row["50_day_ma"] > row["200_day_ma"]:
tesla.loc[index, "Position"] = initial_capital / row["Close"]
# If the 50-day moving average is less than the 200-day moving average, take a short position in Tesla
elif row["50_day_ma"] < row["200_day_ma"]:
tesla.loc[index, "Position"] = -initial_capital / row["Close"]
# Calculate the PnL for the strategy
tesla["PnL"] = tesla["Position"] * tesla["Close"] - initial_capital
# Plot the PnL for the strategy
tesla.plot(x="Date", y="PnL")
This code first imports the necessary libraries, loads the stock data for Tesla, and calculates the 50-day and 200-day moving averages for the stock. It then sets the initial capital for the strategy and initializes the positions for Tesla to 0.
The code then iterates through each row in the dataframe and uses the moving averages to determine whether to take a long or short position in Tesla. If the 50-day moving average is greater than the 200-day moving average, the code takes a long position in Tesla. If the 50-day moving average is less than the 200-day moving average, the code takes a short position in Tesla.
Finally, the code calculates the PnL (profit and loss) for the strategy and plots it using the plot
function.
It is important to note that this is just one example of how a long/short equity strategy for Tesla could be coded, and that other factors, such as risk management and position sizing, may also need to be considered in a real-world implementation. Additionally, this code is for illustrative purposes only and does not constitute investment advice.